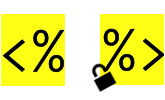
- Don’t turn off request validation unless you need to. Request Validation in ASP.NET explains what this feature does, how to disable it if you must in Web Forms, MVC, and Web Pages and how to manually validate request in absence of it.
- To mitigate Cross-Site Scripting (XSS) attack, encode any input that you output via Response.Write(), embedded code block <%= %> or by setting a property on a control that produces text within a page, using HttpUtility.HtmlEncode() or HttpUtility.UrlEncode(). HttpUtility Methods contains all the encoding and decoding methods for html, html attribute, javascript, query string, and url.
- There is a new embedded code block syntax in ASP.NET 4 that automatically HTML encode output, the <%: %>.
- Use Microsoft AntiXSS Library which extends the built-in encoding methods, provides extra output types like XML, and uses a white-listing approach that defines a list of valid characters (as opposed to the standard .NET framework encoding’s black-listing approach that defines a list of invalid characters). If using .NET 4.x, a version of AntiXSS is already included under System.Web.Security.AntiXss Namespace. You can have the .NET framework use the AntiXSS library by default by registering it via web.config in system.web/httpRuntime, attribute encoderType. See remarks in AntiXssEncoder on how to do this.
- Beginning in .NET 5, a white list based encoder will be the only encoder.
- If you must output certain black listed HTML elements in the input, good practice is to first encode the whole input and then selectively decode those that you wish to output as is.
- Use HttpOnly to protect cookies from being read by client-side scripts, thus prevents being stolen by an XSS attack. You can set it via web.config in system.web/httpCookies element or programmatically using HttpCookie.HttpOnly.
- See OWASP Top 10 for .NET developers part 2: Cross-Site Scripting (XSS).
- Avoid using direct object references such as filenames and database record IDs in your query string, a vulnerability called Insecure Direct Object Reference. Use another key, index, map or indirect method such as GUID for example. If it must be used, make sure user is authorized first.
- See OWASP Top 10 for .NET developers part 4: Insecure direct object reference.
- Never put sensitive information on hidden form fields.
- Never use the Request indexer to access input by name. Be specific when looking for input by using Request.QueryString, Request.Form, Request.Cookies and Request.ServerVariables collections.
- Never change state via a GET request and always check the Request type using HttpRequest.HttpMethod.
- To mitigate Cross-Site Request Forgery (CSRF) attack, one method is to add a token to every form, which is verified when the form is submitted. This process can be automated by implementing an HTTP module. AntiCSRF - A Cross Site Request Forgery (CSRF) module for ASP.NET is a good example of this.
- Do not disable Event Validation as they prevent the falsification of events.
- Do not rely on the Request Headers as they can be easily faked, most notably the Referer Header.
- Do not disable system.web/httpRuntime, attribute enableHeaderChecking in web.config or in code using HttpRuntimeSection.EnableHeaderChecking Property.